View previous topic :: View next topic
|
Author |
Message |
Roshnii
New User
Joined: 30 Sep 2008 Posts: 45 Location: bangalore
|
|
|
|
I have a string of values in the following format
Code: |
Name1#Name2#Y#N#City1#City2#City3#City4#City5# |
The first four values name1, name2, Y flag and N flag will always be at the same first four position. Starting from the 5th Value, there can be 0 to 40 value for the names of the cities.
I tried doing an unstring for the first 4 values to 4 different working storage variables and tried to do a loop for the city names to an array using pointers.
It does not work as Unstring happens only at the first instance and not for the next occurrences of the loop.
Is there any way where unstring can be used to store the city names in an array where the number of cities that can come is not known?
Below is the code that I tried and it did not work.
Code: |
05 WS-REQ-DTL-INP PIC X(200).
05 O-TRY-VAR REDEFINES WS-REQ-DTL-INP.
05 WS-TRY-VAR-ARRAY OCCURS 40 TIMES.
10 WS-TRY-VAR PIC X(05).
PERFORM VARYING WS-SUB FROM 1 BY 1
UNTIL WS-REQUEST = SPACES OR WS-SUB > 40
UNSTRING WS-REQUEST DELIMITED BY '#'
INTO WS-TRY-VAR(WS-SUB), WS-REQUEST
WITH POINTER WS-UNSTR-BEGIN-POSITION
ON OVERFLOW
ADD LENGTH OF WS-TRY-VAR(WS-SUB)
WS-UNSTR-BEGIN-POSITION
ADD 1 TO WS-SUB
END-UNSTRING
END-PERFORM
|
|
|
Back to top |
|
 |
Robert Sample
Global Moderator

Joined: 06 Jun 2008 Posts: 8700 Location: Dubuque, Iowa, USA
|
|
|
|
UNSTRING is not really designed for what you want to do.
One possible way: use UNSTRING into 5 variables -- the first 4 and then a remainder variable. Use reference modification to find the next delimiter in the remainder variable, move the city name to your array, then increment past the delimiter you found and repeat until you don't find any more delimiters. |
|
Back to top |
|
 |
dbzTHEdinosauer
Global Moderator

Joined: 20 Oct 2006 Posts: 6966 Location: porcelain throne
|
|
|
|
you have a table, starting at position 5, with variable item length.
what is the maximum length of city?
add 1 (for the '#' ) and multiply that by 40, giving you the length of the AREA to which you move your string from position 5.
then define your receiving table TABLE as a fixed length table of 40 items,
each item with a max possible length of CITY.
then redefine your TABLE with element fields (each of item length) as ITEM-1, ITEM-2, ...ITEM-40.
now you only need one UNSTRING statement, (delimiting by '#' or space)
UNSTRING AREA DELIMITED BY ... INTO ITEM-1, ITEM-2...,ITEM-40
and no loop.
either spend your time using field declarations, thus requiring only one instruction and no loop
or spend some time figuring out how to write a loop, that won't drive you crazy trying to debug. |
|
Back to top |
|
 |
Rohit Umarjikar
Global Moderator
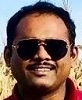
Joined: 21 Sep 2010 Posts: 3077 Location: NYC,USA
|
|
|
|
1.Write a logic to change to ';' between the cities alone
Code: |
Name1#Name2#Y#N#City1;City2;City3;City4;City5; |
2. Unstring step1 rec delimited by '#' into 5 variables
3. Unstring 5th variable delimited by ';' into an array |
|
Back to top |
|
 |
Roshnii
New User
Joined: 30 Sep 2008 Posts: 45 Location: bangalore
|
|
|
|
Hi All,
Thanks for the suggestions.
I used reference modification to get the values to an array as suggested by Robert as UNSTRING does not really work to get values in a loop in a array.
Following is what I used.
Code: |
05 WS-VALUE.
10 WS-NAME1 PIC X(08).
10 WS-NAME2 PIC X(08).
10 WS-Y-FLAG PIC X(01).
10 WS-N-FLAG PIC X(20).
10 WS-CUST-STRING PIC X(200).
05 WS-CUST-TBL.
15 WS-CUST-ARRAY OCCURS 1 TO 40 TIMES.
DEPENDING ON WS-LMT.
20 WS-CUST PIC X(08).
UNSTRING WS-VARIABLE DELIMITED BY '#'
INTO WS-NAME1,
WS-NAME2,
WS-Y-FLAG,
WS-N-FLAG,
WS-CUST-STRING
END-UNSTRING
ADD +1 TO SUB-X
ADD +1 TO SUB-Y
ADD +1 TO SUB-Z
PERFORM VARYING SUB-X FROM 1 BY 1
UNTIL WS-TRNSFR-CUST-VAR(SUB-X:1) = SPACES OR SUB-X > WS-LMT
IF WS-CUST-STRING(SUBX:1) = '#'
MOVE WS-STORE-VAR TO WS-CUST(SUB-Y)
MOVE SPACES TO WS-STORE-VAR
ADD +1 TO SUB-Y
MOVE +1 TO SUB-Z
ELSE
MOVE WS-CUST-STRING(SUBX:1)
TO WS-STORE-VAR(SUB-Z:1)
ADD +1 TO WS-SUB-Z
END-IF
END-PERFORM
|
|
|
Back to top |
|
 |
Rohit Umarjikar
Global Moderator
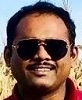
Joined: 21 Sep 2010 Posts: 3077 Location: NYC,USA
|
|
|
|
Did you test? How do you get all the cities into single WS-CUST-STRING, if I understand correctly? |
|
Back to top |
|
 |
Roshnii
New User
Joined: 30 Sep 2008 Posts: 45 Location: bangalore
|
|
|
|
@Rohit Umarjikar
Yes, it is not working when I tested.
The WS-CUST-STRING gets only the first customer and not the entire list.
I tested the loop separately and it works (only if the WS-CUST-STRING has all customers separated by the delimiter).
Can I use an inspect statement to replace all '#' to some other Charater only after the 5th '#' and the do the loop? |
|
Back to top |
|
 |
Rohit Umarjikar
Global Moderator
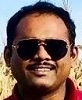
Joined: 21 Sep 2010 Posts: 3077 Location: NYC,USA
|
|
|
|
It seems you have overlooked my earlier suggestion but take a look now and let us see what problems you face then. ahh looks like someone deleted your earlier post. |
|
Back to top |
|
 |
Robert Sample
Global Moderator

Joined: 06 Jun 2008 Posts: 8700 Location: Dubuque, Iowa, USA
|
|
|
|
Why not use this (tested code on Enterprise COBOL 5.1.1)?
Code: |
UNSTRING WS-REQ-DTL-INP
DELIMITED BY '#'
INTO WS-VALUE1
WS-VALUE2
WS-VALUE3
WS-VALUE4
WS-CITIES (01)
WS-CITIES (02)
WS-CITIES (03)
WS-CITIES (04)
WS-CITIES (05)
WS-CITIES (06)
WS-CITIES (07)
WS-CITIES (08)
WS-CITIES (09)
WS-CITIES (10)
WS-CITIES (11)
WS-CITIES (12)
WS-CITIES (13)
WS-CITIES (14)
WS-CITIES (15)
WS-CITIES (16)
WS-CITIES (17)
WS-CITIES (18)
WS-CITIES (19)
WS-CITIES (20)
WS-CITIES (21)
WS-CITIES (22)
WS-CITIES (23)
WS-CITIES (24)
WS-CITIES (25)
WS-CITIES (26)
WS-CITIES (27)
WS-CITIES (28)
WS-CITIES (29)
WS-CITIES (30)
WS-CITIES (31)
WS-CITIES (32)
WS-CITIES (33)
WS-CITIES (34)
WS-CITIES (35)
WS-CITIES (36)
WS-CITIES (37)
WS-CITIES (38)
WS-CITIES (39)
WS-CITIES (40) |
It may not be elegant, but it works and does what you want. You can use a loop after the UNSTRING to find the last city by comparing the city values against spaces. |
|
Back to top |
|
 |
|